- Published on
Empower Your Angular Directives: Handling Multiple Parameters
- Authors
- Name
- Jatin Yadav
@jatiin_yadav
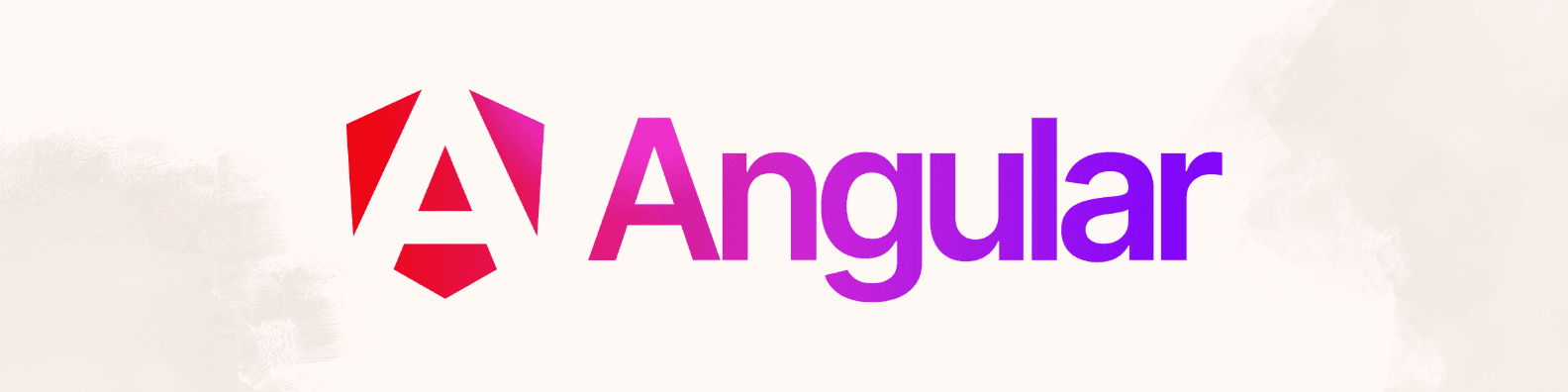
Introduction
Angular, through its toolkit for creating dynamic web applications, offers developers the ability to make directives which enable them make reusable components.
Angular uses directives as its basic constructs, which allow it to extend HTML so that users can define their own tags or syntaxes containing controllers throughout different parts of the application.
In this post we will show you some ways on how you can pass more than one parameter into your directive for Angular applications including some examples like using structural and attribute directives etc.
Using Component Directives
Component directives define custom components which can accept multiple parameters.
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-component-directive',
templateUrl: './component-directive.component.html',
styleUrls: ['./component-directive.component.css']
})
export class ComponentDirectiveComponent {
@Input() firstName!: string;
@Input() lastName!: string;
greetingMessage = ""
ngOnInit() {
this.greetingMessage = `Bonjour!!, Welcome Back ${this.firstName} ${this.lastName}`
}
}
Usage:
<app-component-directive
[firstName]="'John'"
[lastName]="'Doe'">
</app-component-directive>
In app.component.html
, we call the component and pass the inputs firstName
and lastName
. The output on the browser will be:
Bonjour!!, Welcome Back John Doe
Using Attribute Directives
import { Directive, ElementRef, HostListener, Input } from '@angular/core';
@Directive({
selector: '[appAttributeDirective]'
})
export class AttributeDirectiveDirective {
constructor(private elementRef: ElementRef) { }
@Input() colorName!: string;
@Input() opacity!: number;
@HostListener('mouseenter') onMouseEnter() {
this.highlight(this.colorName, this.opacity);
}
@HostListener('mouseleave') onMouseLeave() {
this.highlight('', 1);
}
private highlight(color: string, opacity: number) {
this.elementRef.nativeElement.style.opacity = opacity;
this.elementRef.nativeElement.style.backgroundColor = color;
}
}
Usage:
<div appAttributeDirective [colorName]="'yellow'" [opacity]=0.4>
Hover over me to see the magic!
</div>
In this example we are calling the appAttributeDirective
and passing colorName
as yellow
and opacity
as 0.4
in app.component.html
.
Using Structural Directives
Structural directives are used to add or remove elements from the DOM based on conditions.
import { Directive, Input, TemplateRef, ViewContainerRef } from '@angular/core';
@Directive({
selector: '[appIfHasPermission]'
})
export class IfHasPermissionDirective {
@Input() appIfHasPermission: string;
constructor(
private templateRef: TemplateRef<any>,
private viewContainer: ViewContainerRef
) {}
ngOnInit() {
const userHasPermission = this.appIfHasPermission === 'admin';
if (userHasPermission) {
this.viewContainer.createEmbeddedView(this.templateRef);
} else {
this.viewContainer.clear();
}
}
}
Usage:
<div *appIfHasPermission="'admin'">
<!-- Content to be rendered if user has 'admin' permission -->
<p>Welcome, admin user!</p>
</div>
In this example, the directive appIfHasPermission checks if the user has the admin
permission. If so, it renders the content inside the <div>
. Otherwise, it clears the view container.
Conclusion
- Ensure that you import and declare your directives/components in the appropriate Angular modules to use them across your application.
- For attribute directives and components, you can define as many input properties as needed to pass multiple parameters.
- In each case, you can access the passed parameters using @Input() decorator in the directive/component class.