- Published on
Guide to HashMap Methods in Java
- Authors
- Name
- Jatin Yadav
@jatiin_yadav
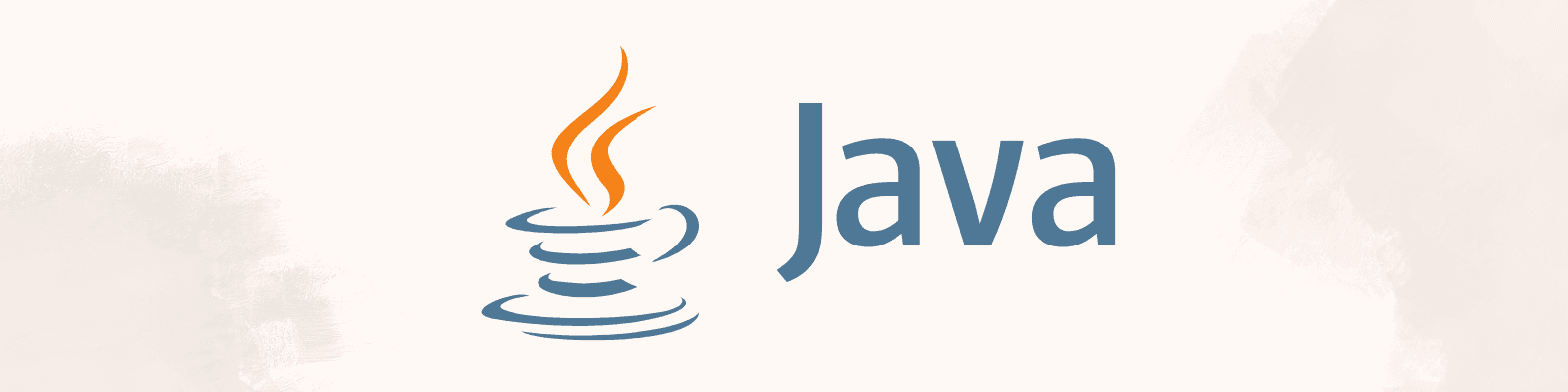
Introduction
A HashMap in Java is a powerful data structure that stores key-value pairs for efficient data retrieval. This guide will walk you through its core methods and how to use them effectively.
Learn how to use HashMap methods to add, retrieve, and manage data efficiently. These methods can make your Java programs faster and easier to maintain.
Explore real-world examples and practical uses of HashMap methods in everyday coding scenarios. By mastering these methods, you'll enhance your problem-solving skills in Java.
1. Basic Methods
- put(key, value): Adds a key-value pair. If the key already exists, the previous value is replaced.
map.put("apple", 10);
- get(key): Retrieves the value associated with the specified key, returns null if key is not found.
Integer value = map.get("apple");
- remove(key): Removes the mapping for the specified key from the map, if present.
map.remove("orange");
- containsKey(key): Checks if the map contains the specified key.
boolean containsKey = map.containsKey("apple");
- containsValue(value): Checks if the map contains one or more keys mapped to the value.
boolean containsValue = map.containsValue(5);
- size( ): Returns the number of key-value mappings in the map.
int size = map.size();
- isEmpty( ): Returns true if the map contains no key-value mappings.
boolean isEmpty = map.isEmpty();
- clear( ): Removes all mappings from the map.
map.clear();
2. Retrieval Methods
- keySet( ): Returns a set of all keys in the map.
Set<String> keys = map.keySet();
- values( ): Returns a collection of all values in the map.
Collection<Integer> values = map.values();
- entrySet( ): Returns a set of all key-value mappings in the map.
Set<Map.Entry<String, Integer>> entries = map.entrySet();
3. Additional Methods
- putAll(map): Copies all mappings from another map into this map.
HashMap<String, Integer> anotherMap = new HashMap<>();
anotherMap.put("banana", 8);
map.putAll(anotherMap);
- getOrDefault(key, defaultValue): Retrieves the value associated with a key, or returns a default value if the key is not present.
Integer value = map.getOrDefault("apple", 0);
- replace(key, value): Replaces the value associated with a key.
map.replace("apple", 20);
- replace(key, oldValue, newValue): Replaces the value associated with a key if it matches the specified old value.
map.replace("apple", 10, 20);
- compute(key, (key, value) -> newValue): Computes a new value for the specified key.
map.compute("apple", (k, v) -> v + 1);
- computeIfAbsent(key, k -> newValue): Computes a new value for the specified key if it is not already mapped.
map.computeIfAbsent("banana", k -> 15);
- computeIfPresent(key, (key, value) -> newValue): Computes a new value for the specified key if it exists in the map.
map.computeIfPresent("apple", (k, v) -> v + 1);
- merge(key, value, (oldValue, newValue) -> resultValue): Merges the specified key and value using a remapping function.
map.merge("apple", 5, (oldValue, newValue) -> oldValue + newValue);
Conclusion
These methods provide a comprehensive toolkit for manipulating and querying data stored in a HashMap in Java, catering to various scenarios from basic CRUD operations to more complex value computations and mappings.